Make the viewController to be open in landscape override the following properties.
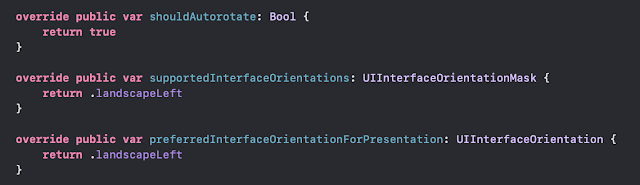
However, it changes the orientation of the presetingViewController. One solution to force the presetingViewController still in portrait is adding the following properties.
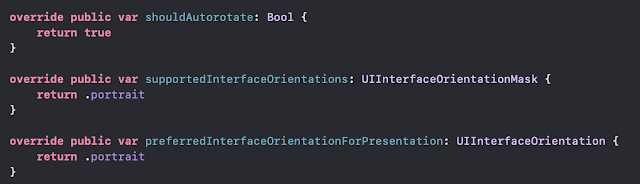